10 Channels Z-Wave Plus v2 and Long Range Certified Example
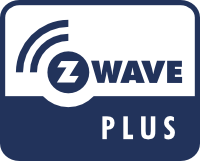
This page contains specific settings option for the certified sketch. All standard Z-Uno parameters and Z-Wave descriptions are listed on this page.
The sketch was certified under the Z-Wave Plus v2 program, certificate ZC14-22030089.
Device represents a complex mains power device with 3 relay switches, 3 LED dimmers, temperature, humidity and motion sensors as well as reed switch.
Z-Uno 1 certified example is locate here.Download Fritzing project
/*
* This scretch was certified by the Z-Wave Alliance.
*
* 3 switches
* 3 dimmers
* 1 motion sensor
* 1 door sensor
* 1 temperature sensor
* 1 humidity sensor
*
*/
#include "ZUNO_DHT.h"// Additional include for DHT sensor support
// Pins definitions
#define LedPin1 A0
#define LedPin2 A1
#define LedPin3 A2
#define LedPin4 PWM1
#define LedPin5 PWM2
#define LedPin6 PWM3
#define MotionPin A3
#define DoorPin 12
#define DHTPin 11
// Switch levels
#define SWITCH_ON 0xff
#define SWITCH_OFF 0
// Global variables for device channels
// switches
byte switchValue1 = 0;
byte switchValue2 = 0;
byte switchValue3 = 0;
// dimmers
byte dimValue1 = 0;
byte dimValue2 = 0;
byte dimValue3 = 0;
// binary sensors
byte lastMotionValue = 0;
byte lastDoorValue = 0;
// temperature & humidity sensor
uint16_t lastHumidityValue = 0;
int16_t lastTemperatureValue = 0;
// Last motion sensor trigger time
uint32_t motionTrigTime;
// enum for some sensors channels, this makes code readable
enum{
SENSOR_MOTION_CHANNEL = 7,
SENSOR_DOOR_CHANNEL,
SENSOR_TEMPERATURE_CHANNEL,
SENSOR_HUMIDITY_CHANNEL,
};
// enum for parameter numbers
enum{
TEMP_HYST_PARAM=64,
HUMIDITY_HYST_PARAM,
MOTION_RETRIGGER_TIME_PARAM
};
// ZUNO_ENABLE setups some global extra build flags
ZUNO_ENABLE(
// LOGGING_DBG // Uncomment for console output on TX0
MODERN_MULTICHANNEL // No clustering, the first channel is mapped to NIF only
MODERN_MULTICHANNEL_S2 // S2 encapsulated NIF in multichannel
MODERN_MULTICHANNEL_S2_ALWAYS );
// Device's endpoints definition
// 3 switch binary
// 3 switch multilevel
// 2 notification sensors
// 2 multilevel sensors
ZUNO_SETUP_CHANNELS(
ZUNO_SWITCH_BINARY(switchValue1, NULL),
ZUNO_SWITCH_BINARY(switchValue2, NULL),
ZUNO_SWITCH_BINARY(switchValue3, NULL),
ZUNO_SWITCH_MULTILEVEL(dimValue1, NULL),
ZUNO_SWITCH_MULTILEVEL(dimValue2, NULL),
ZUNO_SWITCH_MULTILEVEL(dimValue3, NULL),
ZUNO_SENSOR_BINARY_MOTION(lastMotionValue),
ZUNO_SENSOR_BINARY_DOOR_WINDOW(lastDoorValue),
ZUNO_SENSOR_MULTILEVEL(ZUNO_SENSOR_MULTILEVEL_TYPE_TEMPERATURE, SENSOR_MULTILEVEL_SCALE_CELSIUS, 2, 1, lastTemperatureValue),
ZUNO_SENSOR_MULTILEVEL(ZUNO_SENSOR_MULTILEVEL_TYPE_RELATIVE_HUMIDITY, SENSOR_MULTILEVEL_SCALE_PERCENTAGE_VALUE, 2, 1,lastHumidityValue)
);
// Device's configuration parametrs definitions
ZUNO_SETUP_CONFIGPARAMETERS(
ZUNO_CONFIG_PARAMETER_INFO("Temperature hysteresis", "Defines hysteresis of temperature", 1, 20, 5),
ZUNO_CONFIG_PARAMETER_INFO("Humidity hysteresis", "Defines hysteresis of humidity", 1, 20, 5),
ZUNO_CONFIG_PARAMETER_INFO("Motion trigger time", "Minimal trigger interval in ms", 0, 100000, 5000)
);
// Associations of device
ZUNO_SETUP_ASSOCIATIONS(ZUNO_ASSOCIATION_GROUP_SET_VALUE); // Send Basic Set to association group
// Device's S2 keys
ZUNO_SETUP_S2ACCESS(SKETCH_FLAG_S2_AUTHENTICATED_BIT | SKETCH_FLAG_S2_UNAUTHENTICATED_BIT | SKETCH_FLAG_S0_BIT);
// Objects for external periphery
DHT dht22_sensor(DHTPin, DHT22); // DHT sensor
// OS calls setup() function on every device boot
void setup() {
// Configure I/O pins. Analog and PWM will be automatically set up on analogRead/analogWrite functions call
pinMode(LedPin1, OUTPUT);
pinMode(LedPin2, OUTPUT);
pinMode(LedPin3, OUTPUT);
pinMode(LedPin4, OUTPUT);
pinMode(LedPin5, OUTPUT);
pinMode(LedPin6, OUTPUT);
pinMode(MotionPin, INPUT_PULLUP);
pinMode(DoorPin, INPUT_PULLUP);
// Start dht sensor
dht22_sensor.begin();
dht22_sensor.readTemperatureC10(true);
}
// OS calls loop() function repeatedly
void loop() {
// Switches
digitalWrite(LedPin1, switchValue1 == 0);
digitalWrite(LedPin2, switchValue2 == 0);
digitalWrite(LedPin3, switchValue3 == 0);
// Dimmers
analogWrite(LedPin4, ((word)dimValue1)*255/99);
analogWrite(LedPin5, ((word)dimValue2)*255/99);
analogWrite(LedPin6, ((word)dimValue3)*255/99);
// Trigger motion and wait for relax (about 5 sec) before report idle
byte currentMotionValue = digitalRead(MotionPin);
if (currentMotionValue) {
if (!lastMotionValue && ((millis() - motionTrigTime) > zunoLoadCFGParam(MOTION_RETRIGGER_TIME_PARAM))) {
lastMotionValue = 1;
zunoSendReport(SENSOR_MOTION_CHANNEL);
zunoSendToGroupSetValueCommand(CTRL_GROUP_1, SWITCH_ON);
motionTrigTime = millis();
}
} else if (lastMotionValue) {
lastMotionValue = 0;
zunoSendReport(SENSOR_MOTION_CHANNEL);
zunoSendToGroupSetValueCommand(CTRL_GROUP_1, SWITCH_OFF);
}
// Door/Window sensor
byte currentDoorValue = digitalRead(DoorPin);
if (currentDoorValue != lastDoorValue) {
lastDoorValue = currentDoorValue;
zunoSendReport(SENSOR_DOOR_CHANNEL);
}
// Temperature sensor (based on DHT22 digital sensor)
int16_t currentTemperatureValue = dht22_sensor.readTemperatureC10();
if(abs(lastTemperatureValue - currentTemperatureValue) > zunoLoadCFGParam(TEMP_HYST_PARAM)){
lastTemperatureValue = currentTemperatureValue;
zunoSendReport(SENSOR_TEMPERATURE_CHANNEL);
}
// Humidity sensor (based on DHT22 digital sensor)
uint16_t currentHumidityValue = dht22_sensor.readHumidityH10();
if(abs(lastHumidityValue - currentHumidityValue) > zunoLoadCFGParam(HUMIDITY_HYST_PARAM)){
lastHumidityValue = currentHumidityValue;
zunoSendReport(SENSOR_HUMIDITY_CHANNEL);
}
delay(50);
}
Download this sketch
Associations
Device has 2 association groups. See the table below.Endpoint # | Group # | Name | Description | Maximum number of nodes | Commands |
---|---|---|---|---|---|
0 | 1 | Lifeline | Main group for device reports. It's mapped from EP#1 group 1. | 5 | DeviceResetLocally.Report, SwitchBinary.Report |
0 | 2 | User group 02 | Here motion sensor sends Basic on/off commands every time it is triggered. | 5 | Basic.On, Basic.Off |
1 | 1 | Lifeline | Report group. Relay #1 sends report here when it changes value. | 0 | SwitchBinary.Report |
2 | 1 | Lifeline | Report group. Relay #2 sends report here when it changes value. | 0 | SwitchBinary.Report |
3 | 1 | Lifeline | Report group. Relay #3 sends report here when it changes value. | 0 | SwitchBinary.Report |
4 | 1 | Lifeline | Report group. Dimmer #1 sends report here when it changes value. | 0 | SwitchMultilevel.Report |
5 | 1 | Lifeline | Report group. Dimmer #2 sends report here when it changes value. | 0 | SwitchMultilevel.Report |
6 | 1 | Lifeline | Report group. Dimmer #3 sends report here when it changes value. | 0 | SwitchMultilevel.Report |
7 | 1 | Lifeline | Report group. Motion sensor sends report here when it triggers. | 0 | Notification.Report |
8 | 1 | Lifeline | Report group. Door sensor sends report here when it triggers | 0 | Notification.Report |
9 | 1 | Lifeline | Report group. Temperature sensor sends report here when its value changes greater then hysteresis defined by parameter #64. | 0 | SensorMultilevel.Report |
10 | 1 | Lifeline | Report group. Humidity sensor sends report here when its value changes greater then hysteresis defined by parameter #65. | 0 | SensorMultilevel.Report |
Basic command class interoperability
Below is listed command class basic mapping for all the endpoints.# Endpoint | Mapped command class | Values |
---|---|---|
0 | SwitchBinary | 0 means OFF, 0xFF (255) - ON |
1 | SwitchBinary | 0 means OFF, 0xFF (255) - ON |
2 | SwitchBinary | 0 means OFF, 0xFF (255) - ON |
3 | SwitchBinary | 0 means OFF, 0xFF (255) - ON |
4 | SwitchMultilevel | 0 means OFF, 1 - 99 - dimmer value. |
SwitchMultilevel | 0 means OFF, 1 - 99 - dimmer value. | |
6 | SwitchMultilevel | 0 means OFF, 1 - 99 - dimmer value. |
7 | – | – |
8 | – | – |
9 | – | – |
10 | – | – |
Configuration parameters
In addition to the Z-Uno system configuration parameters this sketch provides user-side configuration parameters. See the table below.# | Description | Size | Default value | Possible values |
---|---|---|---|---|
64 | Temperature hysteresis for sensor reports. This parameter is defined in 10th of degree. | 4 bytes | 5 | 1–20 |
65 | Humitity hysteresis for sensor reports. This parameter is defined in 10th of percent. | 4 bytes | 5 | 1–20 |
66 | Motion sensor's retrigger interval in milliseconds | 4 bytes | 5000 | 0–100000 |
S2 Levels
This sketch supports these S2 keys:
- S0
- S2_UNAUTHENTICATED
- S2_AUTHENTICATED
Notification types & events
# Endpoint | Notification Type | Notification Events |
---|---|---|
7 | NOTIFICATION_TYPE_SECURITY = 0x07 | NOTIFICATION_EVENT_MOTION_DETECTION_UL = 0x08 |
8 | NOTIFICATION_TYPE_ACCESS_CONTROL = 0x06 | NOTIFICATION_EVENT_WINDOW_DOOR_OPENED = 0x16 NOTIFICATION_EVENT_WINDOW_DOOR_CLOSED = 0x17 |