Humidity and temperature sensor DHT11
This sketch shows how to connect DHT11 sensor to the Z-Uno board and periodically report humidity and temperature values to channels Sensor Multilevel.Download Fritzing project
// demo sketch for connecting DHT11 humidity and temperature sensor to Z-Uno
// add library
#include "ZUNO_DHT.h"
// pin connection
#define DHTPIN 9
DHT dht(DHTPIN, DHT11);
int humidity; // here we will store the humidity
int temperature; // here we will store the temperature
// set up channels
ZUNO_SETUP_CHANNELS(
ZUNO_SENSOR_MULTILEVEL_TEMPERATURE(getterTemperature),
ZUNO_SENSOR_MULTILEVEL_HUMIDITY(getterHumidity)
);
void setup() {
dht.begin();
Serial.begin();
Serial.println("start");
}
void loop() {
// obtaining readings from the sensor DHT
humidity = dht.readHumidity();
temperature = dht.readTemperature();
Serial.print("Humidity = ");
Serial.print(humidity);
Serial.print(" % ");
Serial.print("Temperature = ");
Serial.print(temperature);
Serial.println(" *C");
// send data to channels
zunoSendReport(1);
zunoSendReport(2);
// send every 30 second
delay(30000);
}
byte getterTemperature() {
return temperature;
}
byte getterHumidity() {
return humidity;
}
Download this sketch
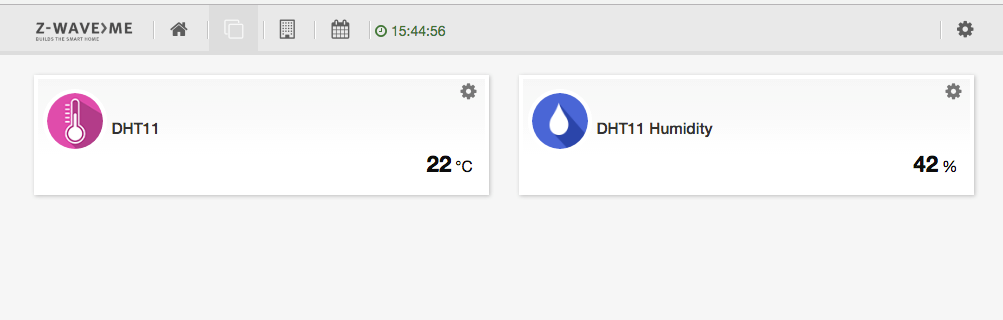
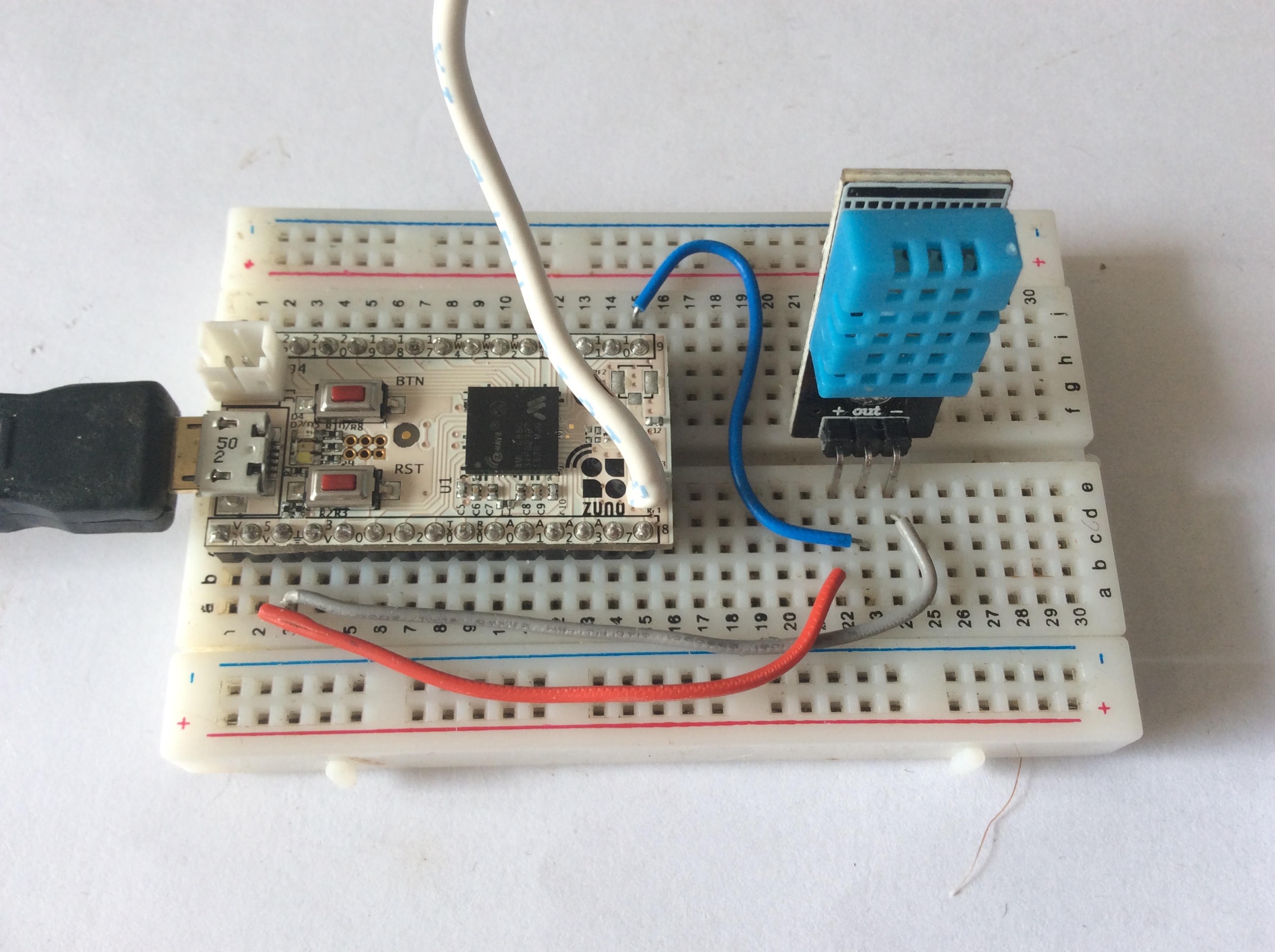